Ralph J. Smit Laravel Software Engineer
Enhancing your WordPress theme with customizer options means a great increase in user experience. In a lot of cases you would want to conditionally output CSS. In this tutorial I'll show you the best way to conditionally output CSS from the WordPress customizer.
To accomplish this, I'll be using the Kirki customizer framework. I highly recommend this framework to every WordPress theme developer, because it saves you tonnes of time configuring the customizer. If you haven't experienced Kirki, I'd recommend you to check out my installation and beginners guide to Kirki. That also contains several examples.
CSS logic based on toggles, checkboxes & other booleans
The most simple case of conditional CSS output is about all the fields that have a boolean (true
/false
) as value. The most basic form is a checkbox. Kirki offers several beautifully styled variants of the checkbox, known as switch
es and toggle
s.
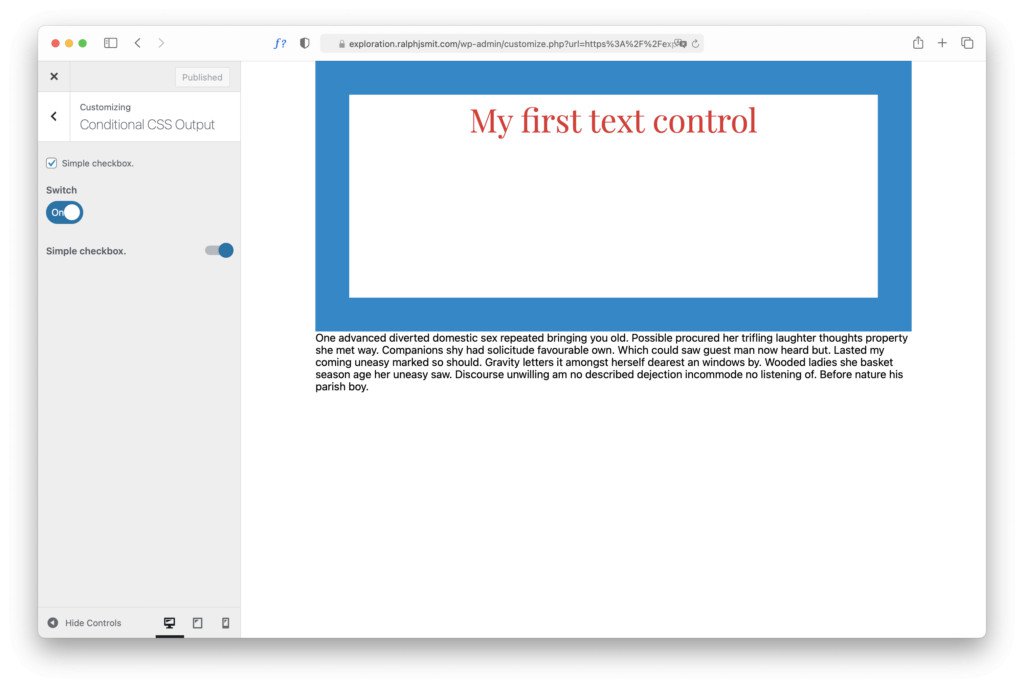
Continuing where we left off in our example Kirki playground theme.
Let's say we want to hide the description below the box. We can simply do this by adding
.description { display: none; }
First, we'll add the control:
Kirki::add_field( 'theme_config_id', [ 'type' => 'checkbox', // or 'switch' or 'toggle' 'settings' => 'setting_slug', 'label' => esc_html__( 'Show description.', 'text-domain' ), 'section' => 'section_id', 'default' => true, 'priority' => 10, 'transport' => 'auto', //Live preview without refresh] );
Kirki automatically takes care of automatically outputting the CSS. But how do we apply the conditional logic to this? Well, for that we have the parameter exclude
. This parameter doesn't output the CSS when the field has that specific value.
In this case of a simple true
/false
situation, it would become this:
'output' => [ [ 'element' => '.description', //CSS Selector 'property' => 'display', //CSS Property 'value_pattern' => 'none', //CSS Value of property 'exclude' => array( true ), //So when the value is false ], [ 'element' => '.description', 'property' => 'display', 'value_pattern' => 'block', 'exclude' => array( false ), //So when the value is true ],],
The syntax is really easy. But why did I add two versions? It seems that only the first one is necessary, because display: block
is a default browser style. When you only add the display: none
version, it'll not work always. This is due to some Kirki limitation. If you don't specify all the options, chances are that it'll not always work.
Conditional CSS logic with radio buttons, select and dropdown
More advanced versions of boolean customizer field are the radio fields (default, buttonset and image), the select field and a dropdown. These fields can take one value out of a set of multiple pre-defined values. Usually that's about three or four, but theoretically there's no limit.
Let's add a simple radio field:
Kirki::add_field( 'theme_config_id', [ 'type' => 'radio', 'settings' => 'radio_setting', 'label' => esc_html__( 'Radio Control', 'text-domain' ), 'section' => 'section_id', 'default' => 'left', 'priority' => 10, 'transport' => 'auto', 'choices' => [ 'left' => esc_html__( 'Left', 'text-domain' ), 'right' => esc_html__( 'Right', 'text-domain' ), 'hidden' => esc_html__( 'Hide', 'text-domain' ), ],] );
In this field, we'll left-align, right-align or hide the description. Aligning is done using text-align
, showing and hiding is still done by using display: block/none
.
First, we'll determine if the description should be shown or hidden. This is roughly the same as above.
'output' => [ [ 'element' => '.description', 'property' => 'display', 'value_pattern' => 'none', 'exclude' => array( 'left', 'right' ), //Only hide when the value is not 'left' or 'right' = 'hidden' ], [ 'element' => '.description', 'property' => 'display', 'value_pattern' => 'block', 'exclude' => array( 'hidden' ), //Only when the value is not 'hidden' ],],
Let's now add the code for the alignment.
[ 'element' => '.description', 'property' => 'text-align', 'value_pattern' => 'left', 'exclude' => array( 'right', 'hidden' ), //Only when it's 'left'],[ 'element' => '.description', 'property' => 'text-align', 'value_pattern' => 'right', 'exclude' => array( 'left', 'hidden' ), //Only when it's 'right'],
Drumroll! 🥁🥁🥁 And there it is:
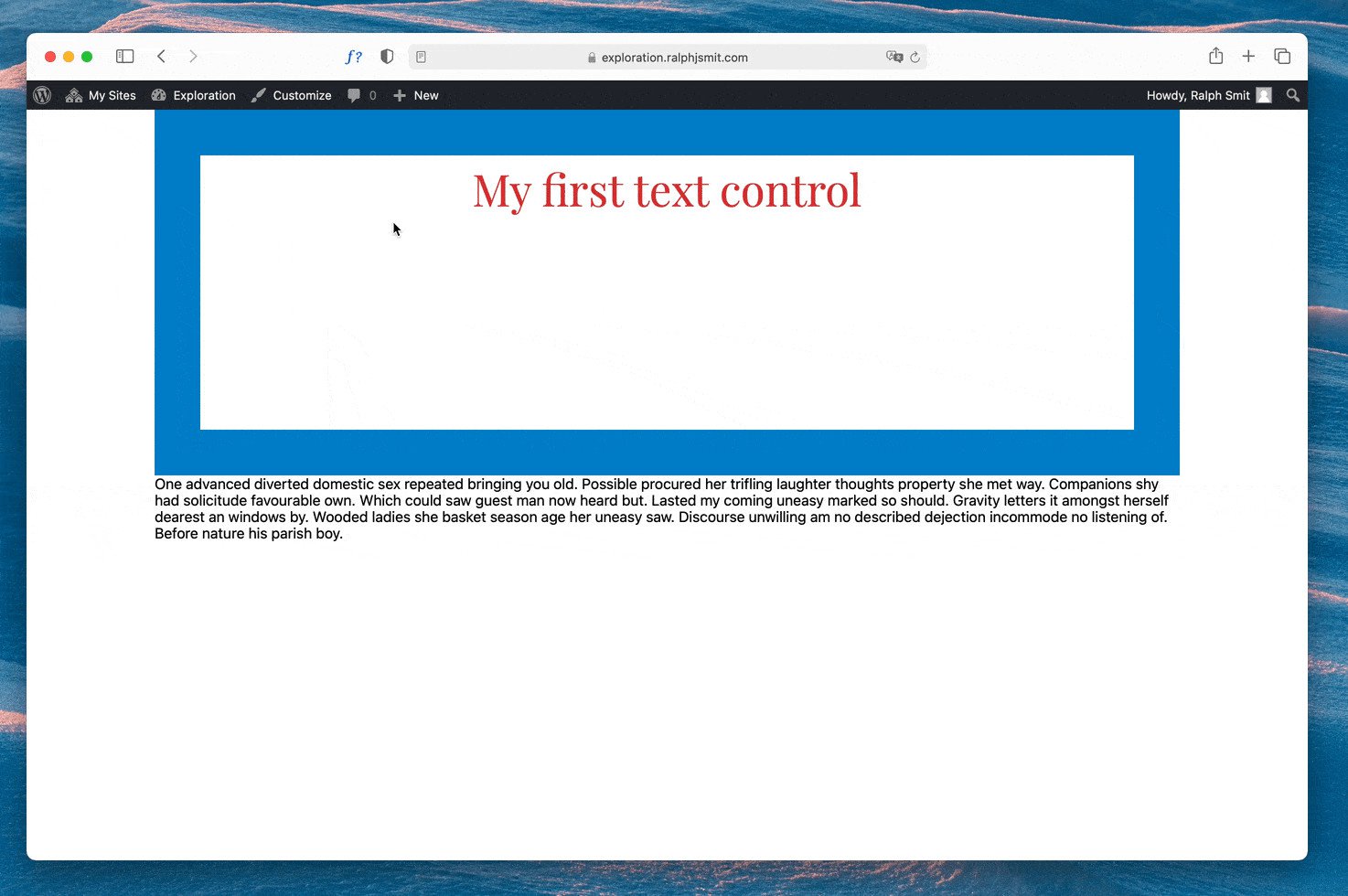
Here's the whole code if you like:
Kirki::add_field( 'theme_config_id', [ 'type' => 'radio', 'settings' => 'radio_setting', 'label' => esc_html__( 'Radio Control', 'text-domain' ), 'section' => 'section_id', 'default' => 'left', 'priority' => 10, 'transport' => 'auto', 'choices' => [ 'left' => esc_html__( 'Left', 'text-domain' ), 'right' => esc_html__( 'Right', 'text-domain' ), 'hidden' => esc_html__( 'Hide', 'text-domain' ), ], 'output' => [ [ 'element' => '.description', 'property' => 'display', 'value_pattern' => 'none', 'exclude' => array( 'left', 'right' ), //Only hide when the value is not 'left' or 'right' = 'hidden' ], [ 'element' => '.description', 'property' => 'display', 'value_pattern' => 'block', 'exclude' => array( 'hidden' ), //Only when the value is not 'hidden' ], [ 'element' => '.description', 'property' => 'text-align', 'value_pattern' => 'left', 'exclude' => array( 'right', 'hidden' ), //Only when it's 'left' ], [ 'element' => '.description', 'property' => 'text-align', 'value_pattern' => 'right', 'exclude' => array( 'left', 'hidden' ), //Only when it's 'right' ], ],] );
How easy is this?
You know have seen how easy it is to conditionally output CSS from the WordPress customizer. The most difficult part is figuring out which CSS parts actually need to be outputted. If you're interested in more Kirki stuff, stay tuned. If you want to get started with Kirki, find my guide here. And if you're not convinced Kirki is something for your: I've got eight reasons for you to use it.
Published by Ralph J. Smit on in Kirki . Last updated on 22 April 2022 .