Ralph J. Smit Laravel Software Engineer
Laravel is a great framework for rapid application building. It allows you to easily connect to a database. If you're developing locally, chances are that you need to confirm that the application is connected to a database, for example when you're debugging something.
In this tutorial I'll show you how to check which database your Laravel app is connected to.
There is a simple code snippet to check the name of the current database connection, and if not, it will return 'none'. There are two ways to it:
-
Place it somewhere in a Blade template or PHP-file (recommended for one-time debugging)
-
Place it in a random file and
dump()
it to the dump-server (recommended for all other use cases)
Echo the Laravel database name in Blade/PHP
The simplest way it to place the following script in a Blade or PHP file. This will output the name of the database or return 'none' if there is no connection.
<strong>Database Connected: </strong><?php try { \DB::connection()->getPDO(); echo \DB::connection()->getDatabaseName(); } catch (\Exception $e) { echo 'None'; }?>
If you view it in the browser, it gives you the name of the connected database.
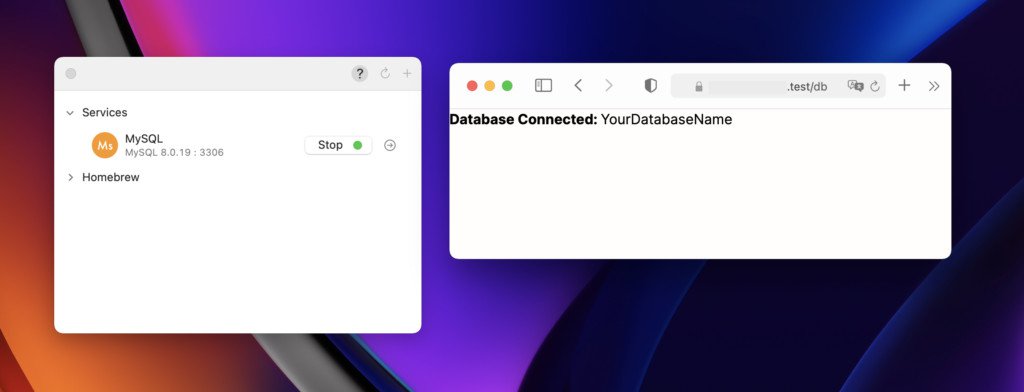
Checking whether the application is connected to a Laravel database.
Using the dump-server to check this
But it is not always handy to show this immediately on the front-end. You can remedy that by using the php artisan dump-server
. This allows you to log the database connection to your Terminal / Command Line Tool, whilst not displaying it in the browser.
First, start the dump server. Do this by opening your CLI, look Terminal, iTerm2 or an other. Personally I prefer iTerm2, but the default terminal is also a good choice if you don't need a CLI often. Run this code to start the dump server:
php artisan dump-server
Next, place the following code somewhere in your files. You could still place it in Blade or PHP file, but also in a controller or the boot()
function of the /app/Providers/AppServiceProvider.php
file. Personally I recommend placing it in the boot()
method.
try { \DB::connection()->getPDO(); dump('Database connected: ' . \DB::connection()->getDatabaseName());} catch (\Exception $e) { dump('Database connected: ' . 'None');}
Note: if you place the above code in a PHP or Blade files, make sure to wrap it in PHP tags: <?php
and ?>
.
If you then load a page, you see that your CLI automatically shows you which database it is connected to. 🚀
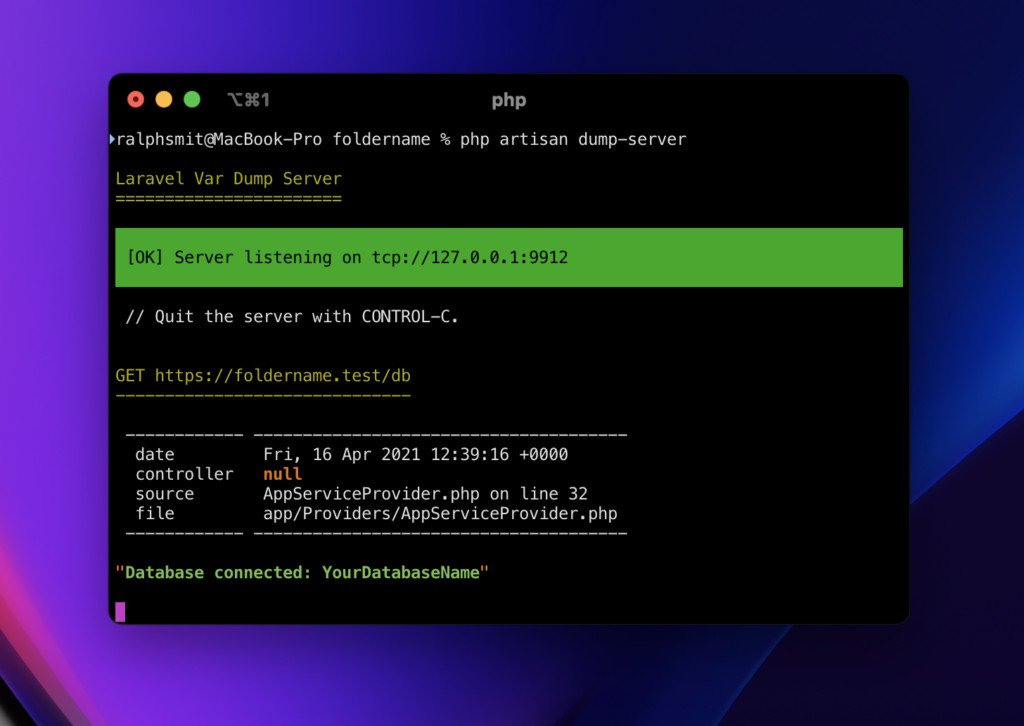
Using the php artisan dump-server
command to check the database connection.
Conclusion
As you see, it's very easy to confirm that your Laravel app is connected to a database. I especially use this for my local apps. Let me know in the comments how it goes 👇
Check out my other articles and learn how to set set up local MySQL databases for Laravel and see how to set up Tailwind CSS & SASS with Laravel Mix.
Published by Ralph J. Smit on in Laravel . Last updated on 10 March 2022 .