Ralph J. Smit Laravel Software Engineer
When I was busy moving my WordPress-blog to Laravel, of course I also considered the SEO-aspect of the site. I googled around for some Laravel-SEO packages, but most of them had a nice API (think: seo()->title(/** */)->twitter()
), but didn't do much automatically. You still had to call those methods and input the right stuff.
I've always enjoyed working with Spatie's MediaLibrary package, which basically associates media files with Eloquent models of your choice (morph). This would be a very good strategy for SEO as well, I thought. Think of associating a SEO-model with a Post model.
The second thing that this package does is providing you with a lot of sensible default tags. You'll only need to fill in the configuration file once, and the package will generate all the default tags you need.
You can find the package here.
Installing the SEO package
Installing the package can be done via composer. Also, publish the migrations and the config file:
composer require ralphjsmit/laravel-seo php artisan vendor:publish --tag="seo-migrations"php artisan vendor:publish --tag="seo-config"
Next, you should go to the config file (seo
) and fill in every setting.
The final step is to add the following in your Blade-layout file and output that on every page:
{!! seo() !!}
Associating Eloquent models
For the following example, I'll assume that we have a Post
model with SEO:
use RalphJSmit\Laravel\SEO\Support\HasSEO; class Post extends Model{ use HasSEO; // ...}
Now, every time a new model will be created, it will automatically get a SEO model assigned. On that SEO model, you can update the title
, description
, author
and image
properties:
$post->seo->update([ 'title' => 'My title for the SEO tag', 'image' => 'images/posts/1.jpg', // Will point to `public_path('images/posts/1.jpg')`]);
You can also dynamically fetch properties from the $post
model itself, without having to keep the related SEO-model in sync:
// Add this on your Post class.protected function getDynamicSEOData(): SEOData{ return new SEOData( title: $this->title, description: $this->excerpt, author: $this->author->fullName, );}
There are many dynamic properties to override, the best thing is to take a look at the docs for all the properties.
Outputting the SEO-model on the page
Now, at this point you might be wondering how the package knows which SEO model it should use to generate tags on the page. To tell the package the associated SEO-model, you can pass it to the seo()
function:
{!! seo()->for($post) !!}{{-- Or pass it directly to the `seo()` method: --}}{!! seo($post ?? null) !!}
This will output all the meta-tags for the SEO-model, plus the default site tags. If you don't pass a SEO-model, it will only output the default site tags.
Filament plugin
The package also has a dedicated simple plugin for Filament PHP, the greatest package for TALL admin panels and forms.
You can view the Filament package here.
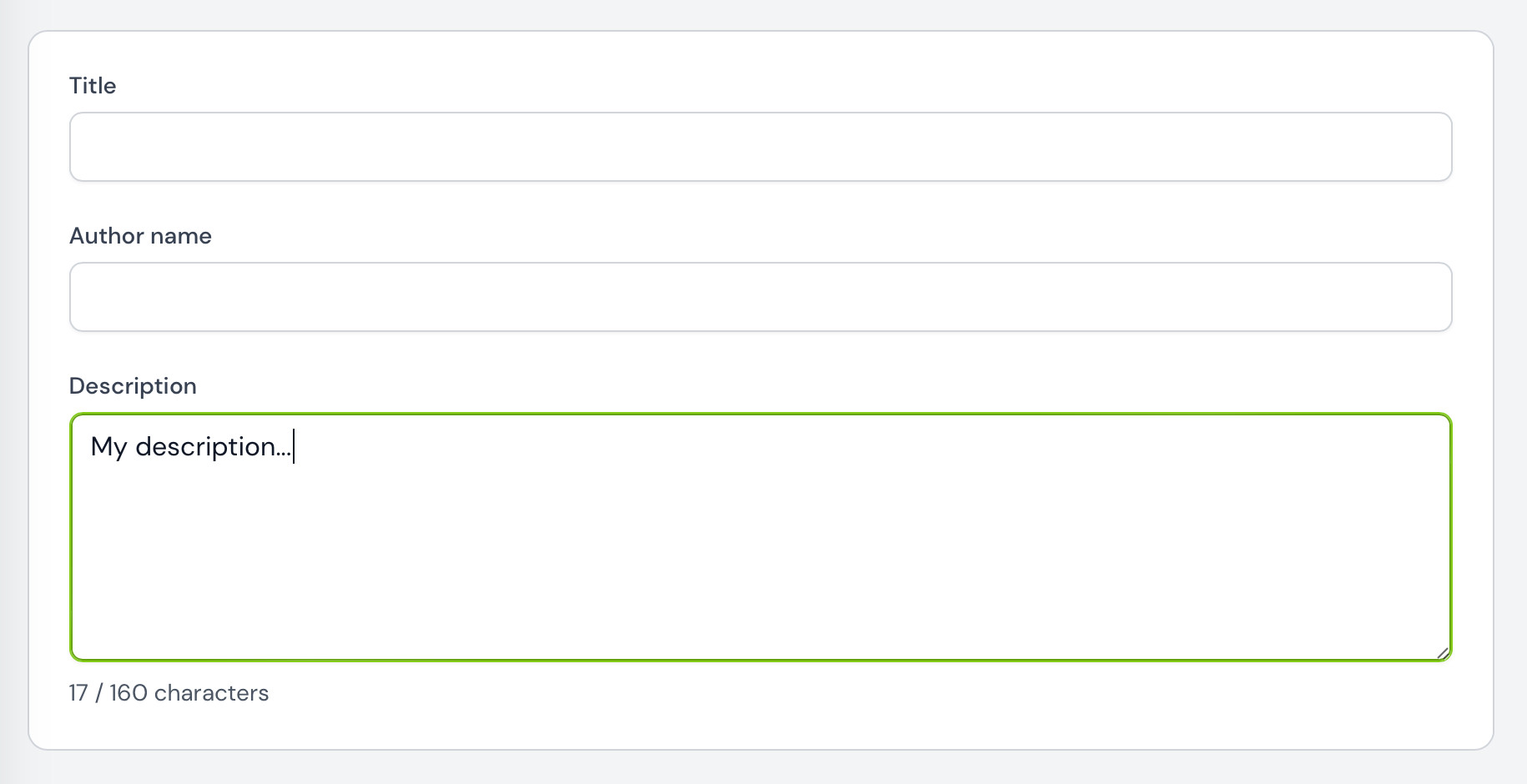
Thanks
Thanks for reading! I really appreciate it. This is already my sixth or seventh Laravel-package and I still enjoy creating them.
The package works best on new projects and I really hope you will benefit from it as well.
You can find (and star😜) the repo on GitHub. Feel free to open an issue or leave a comment with your ideas and questions!
Published by Ralph J. Smit on in Laravel . Last updated on 26 April 2022 .