Ralph J. Smit Laravel Software Engineer
When developing WordPress themes that use the Elementor page builder, you'll sometimes want to add additional CSS to the stylesheet of a page. Elementor provides an extremely large amount of functions and hooks, but examples are scarce. In this article, I'll give an example of adding CSS to an Elementor stylesheet.
How do CSS stylesheets work in Elementor
For this tutorial, I created a very simple page in Elementor. This page only contains a title. I didn't change any styling, but only added a 64px margin. If you open the page in Firefox (I use the Developer Edition), you can easily view the styles by going to Style Editor. At the left (or top, depending on the width of the inspect pane), you'll see a list of each CSS file.
Elementor creates CSS files with the following naming structure: post-X.css. In this case, we have both post-4.css
and post-37.css
.
I see that post-4
contains some general CSS. In post-37
I see the margin I added. So post-37.css
is the current stylesheet.
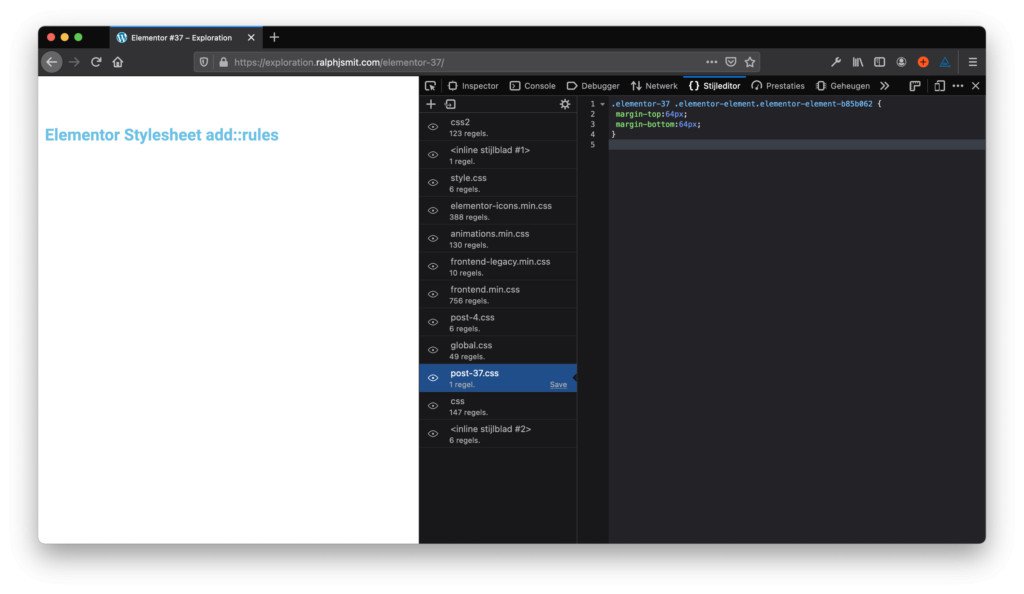
CSS stylesheets on an Elementor page.
Adding rules to this stylesheet
Let's now add a CSS rule to this stylesheet. Let's change the background color of the page using our own function. The Element has the following HTML structure. Here the number 37
also comes back again as the elementor-id
(the prefix data-
is needed for custom attributes).

HTML structure of an Elementor page. This <div>
is outputted by the the_content()
function on pages made with Elementor.
Let's apply the following CSS:
.elementor-37 { background-color: #0077ff; }
Step 1 – Load a separate PHP-file to keep everything structured
We'll place our Elementor code in a separate PHP file. This keeps the theme structured and makes sure the functions.php
will not become cluttered.
Create a file called elementor.php
. Go to your functions.php
and include it:
//Check if Elementor is activeif (class_exists('Elementor')) { locate_template('elementor.php', true, true);}
We first check if the class exists (so that the plugin is active and works), otherwise loading the file is pointless (and we'll get PHP errors as a result).
Step 2 – Structuring the elementor.php
We'll use a function that adds the CSS to the stylesheet. That function should be called on the right moment. That moment is when the stylesheet itself is being generated.
That moment has a hook: elementor/element/parse_css
. When that hook is called, we'll execute our function with content. We connect the hook to the function by using add_action()
.
add_action('elementor/element/parse_css', function( $post_css, $element ) { //}
Step 3 – Get the stylesheet
Now that we're at the right moment when the stylesheet is generated, we'll
-
get that stylesheet; and
-
add our extra CSS rules.
$post_css->get_stylesheet()->add_rules();
The add_rules()
function takes three parameters:
Name | Type | Required | Description |
$selector | string | Required | CSS selector. |
$style_rules | array or string | Optional | Style rules. Default is null . |
$query | array | Optional | Media query. Default is null . |
The parameters for the Elementor Stylesheet::add_rules
function. Source: Elementor Code Reference.
As you see, we'll need at least two parameters to get a sensible CSS rule.
.elementor-37 { background-color: #0077ff; }
The CSS rule above translates into:
$selector = '.elementor-37'; $style_rules = [ 'background-color' => '#0077ff', //property and value];
This makes:
$post_css->get_stylesheet()->add_rules($selector, $style_rules);
And... 🤯 💥boom!
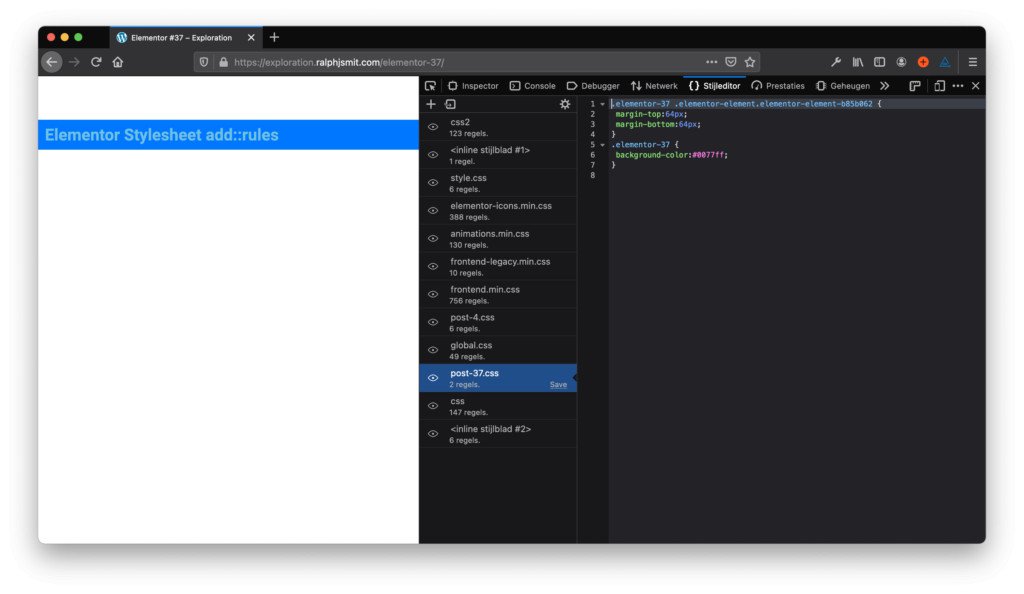
Our styling is added in the post-37.css
file by Elementor.
If you don't immediately see the changes, go to Elementor > Settings > Tools > Regenerate CSS. Elementor will recreate each CSS file. Making a change to a page and saving that is also sufficient.
You could also apply the color to the <body>
. In this way, you can apply CSS on specific pages or Elementor widgets by using simple PHP logic. You could use the customizer to add theme options, get them with PHP, apply some logic and output the CSS.
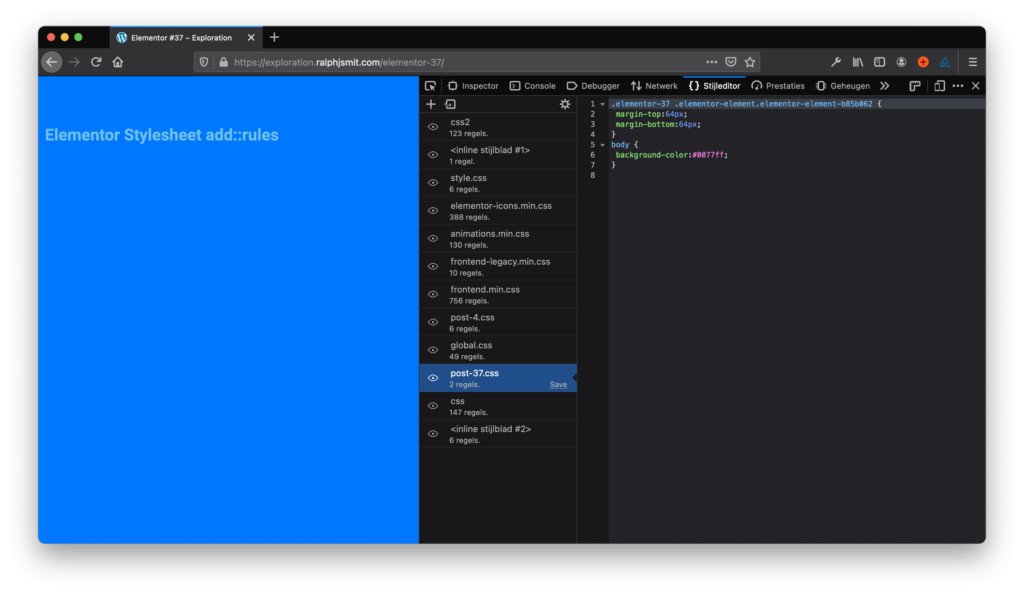
The total code comes down to the following:
add_action('elementor/element/parse_css', function( $post_css, $element ) { $selector = 'body'; $style_rules = [ 'background-color' => '#0077ff', //property and value ]; $post_css->get_stylesheet()->add_rules($selector, $style_rules, null); // Repeat for every rule }, 10, 2 );
How to use media queries?
The third parameter, $query
, contains the media queries that should be applied. As adding media queries is a profession in its own right, I've written about that in a follow-up post.
Published by Ralph J. Smit on in Elementor . Last updated on 12 March 2022 .